Divide and conquer. Develop your application as a series of simpler, dedicated purpose programs, each in the language appropriate to the task
Less code, Better code, Smaller code
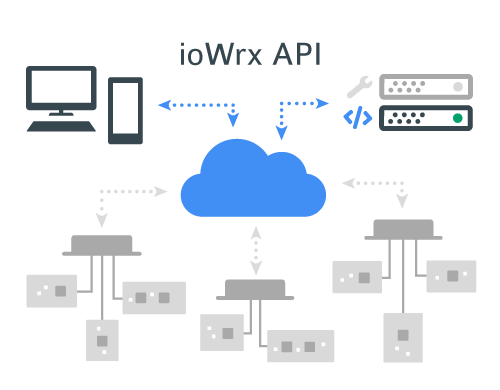
Developers use the ioWrx API* to write applications that leverage ioPanel, ioBlok and the ioWrx IMS.
The ioWrx API is designed to give a developer three important benefits:
- less code - by leveraging ioWrx Capabilities
- better code - by providing I/O and network abstraction
- simpler code - by allowing a larger programming task to be broken down into simpler, independent programs, each in the language best suited to the task
Develop embedded online
By leveraging network abstration, the ioWrx API allows most software development to be done "out of the box", meaning on more powerful development servers with desktop development tools.
For example, the ioWrx API allows a C language control program to be developed on a secure VPS, with the Eclipse IDE and debugged with a local GNU debugger, while interacting with live I/O on a remote ioBlok.
No cross-compilation or remote debugging cables are required during development.
In addition to speeding code/test cycles, such remote development opens the door to collaboration across development teams.
ioWrx API Capabilities - Less code

You can look at the ioWrx API as code that doesn't need to be written.
Even the most basic ioWrx programs,
such as the python script shown above,
begin life which a rich set of built-in capabilities,
hidden behind the simple call to Init()
.
ioWrx capabilities are easy to use. Not only do they require little or none of your own code to implement, they are generally configured through text files consisting of simple directives. This helps keep information that changes between installations – like the IP address of specific computers, the timezone or the type of debug information being traced – out of your code.
ioWrx Capabilities were designed with control applications in mind. They provide a toolbox of built-in functionality including:
- software component installation and updates
- log file generation and uploading
- hardware and OS self monitoring
- visual editing of I/O names, types and reporting controls
- automatic network discovery
- automatic recognition and configuration of ioPanels
- DHCP and NTP self configuration
- Client and Host AP WiFi
- Watchdog Timer
- UI web server
An overview of ioWrx capabilities can be found here.
ioWrx API I/O and Network Abstraction - Better code
The ioWrx API allows developers to write control programs at a higher level of abstraction, that aren't encumbered with hardware implementation or networking details.
For example, an ioWrx control C++ program can use ioWrx API calls like this:
iowrx.SetIOOutputState(“FanSpeed”, “High”)
This program would not require code specifying which bits in what ioPanel must switch to make the fan speed high, nor would it contain code specifying the IP address of the ioPanel which controls the fan speed, nor code to communicate with it.
ioWrx handles I/O and network implementation details as configuration data, which reside outside of application code. The resulting control program could be used with different ioPanels or ioBlok network configurations, which permits such decisions to be made at the time of installation, or at the time of production, in the case of a control system shared across a product line with multiple related models.
Simpler code
With ioWrx's network and I/O abstraction, it is often possible and desirable to write applications as a number of smaller cooperating programs, each handling a specific task, running at the location where it is needed, and written in the programming language best suited to that task.
For example, an operator interface for the above control program might be implemented as a web interface, and contain the following javascript code to display the current state of the fan speed:
//get the current state info and display itioWrx.GetIOLastReportedState(selectedNode.name, function(data){ if ( data.result ) { dispHTML = "<tr> <td>State Name</td> <td>"+data.strings[0]+"</td> </tr>"; } else console.log(data);});
In this example, not only does the ioWrx API provide abstraction of the I/O, but it implicitly connects different parts of the application, through a shared and synchronized view of the I/O.
Further extending the above example, a third program, written in python and running on a server, might react to the change in fan speed an log an entry in a MySQL database. The ioWrx API makes it possible to write applications in different programming languages, that work together across networks, as if I/O were local.
Event driven
In addition to network and I/O abstraction, ioWrx provides I/O event monitoring, which allows ioWrx programs to focus on handling events, rather than detecting them.
Through the ioWrx API, ioPanels are configured to handle real-time tasks such as polling inputs for changes in state, or key presses or transitions through threshold levels. Developers need only write code that handle the resulting reported activity.
Shared variables
ioWrx event handling is not limited to I/O events, but is extended to application-defined information too, called Schema Variables. One ioWrx program can declare such a Schema Variable, and another ioWrx program elsewhere on the network can define code to react to changes in its value.
ioWrx Schema Variables provide a powerful tool for application development, allowing for the compartmentalisation of application behaviour. For example, control logic can be implemented as a collection of separate, stand alone algorithms, each having its own "do this when that happens" logic.
User interfaces can be then programmed independently of control logic, making it possible to combine different user interface implementations with the same program logic.
The ioWrx API abstracts all of the language and networking interfacing implied in the above scenarios.
Network fault detection and handling
ioWrx further extends event handling to allow monitoring of network and hardware faults. In addition to monitoring events related to application I/O, the ioWrx API generates connectivity events, allowing applications to detect and respond to the failure or disconnection of individual ioPanels or the loss of connectivity to individual ioBloks.
This allows the development of robust networked applications which can pin point network connectivity problems.
Timer and task scheduling
In any development environment, managing multiple tasks in parallel is a challenge. Behind the scenes, the ioWrx API provides the management of all I/O processing tasks, including inter-application messaging. But ioWrx also provides three different approaches for extending multitasking to user-defined tasks:
-
Timers
- ioWrx Timers allow developers to define tasks that must occur either periodically, or only after a timed intervals. Timers are great for implementing time outs on user interaction, or to respect the delays implicit in the operation of machinery.
-
User Tasks
- ioWrx User Tasks allows low priority or long running tasks to be divided into smaller incremental steps, to maintain responsiveness of the overall application.
-
GUI processing
- Finally, perhaps viewed as the inverse of User Tasks, the ioWrx API also allows ioWrx processing itself to be handled in the background of larger real-time programming environment. This multitasking model is often required to adapt to some graphical user interface programming environments, for example Visual Basic or Qt user-interfaces, which may hide the main processing thread.